BIRT currently supports many data sources, including XML, Web Services, JDBC and flat files. This list can also be extended by implementing BIRT extension points. Using this method is called implementing an ODA(Open Data Access) extension.
An ODA allows the developer to add custom GUI elements to the BIRT designer to create and manipulate a data source. This method is described in a set of articles located on BIRT-Exchange. BIRT also supplies a scripted data source that allows a developer to write code in a simplified interface to retrieve data using Java or JavaScript. This post explains how a scripted data source can be implemented to retrieve XLS data using the Source Forge Java Excel API project. For more information on using this API see this blog post.
Adding the JAR to the BIRT Project
First download the Java Excel API and extract the jxl.jar file from the download. You can add this Jar to your BIRT project in several different ways. See this post for designer classpath options. One simple way to add the jar is to put the jar in your BIRT Project Resource folder and then add a reference to it in the report design. You can set the resource folder in the BIRT Designer Preferences (Window-Preferences-Report Design-Resource). The resource folder is also a web.xml setting in the deployed viewer.
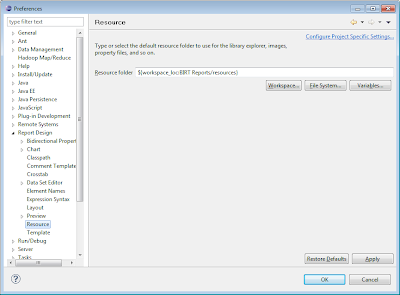
In the above screen shot we have a resources folder under the BIRT reports project. Just import the jxl.jar into this folder. To create the reference to the jar in a report design, select the properties view for the report and click on resources. Click the add file button in the jar files section of the report resources and add the jar.
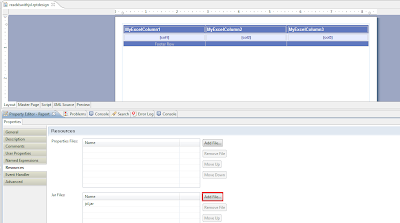
The jar file should now be accessible in the designer from script or expressions. When you deploy the report to the Viewer make sure to copy the jar to the resource folder of the deployed Viewer.
If this method of adding the jar is not desirable you can always create a folder in the project containing the lib and add the jar to the project classpath entry (Window-Preferences-Report Design-Classpath). This will allow the jar to be accessed at design time. When deploying the report you can then just copy the jar to the WEB-ONF/lib folder of the deployed viewer.
Implementing the Scripted Data Source/Set
BIRT supports implementing a scripted data source in Java or JavaScript. In this example we implement it using JavaScript. Although the code is written in JavaScript we can still call Java code by using the importPackage method. This example uses this approach.
Use the new data source wizard to add a scripted data source and use the new data set wizard to add a new scripted data set. In this example we are reading a three column xls file, so we define 3 columns in our dataset.
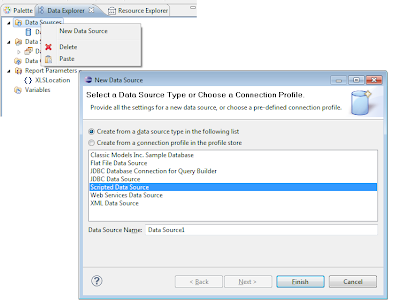
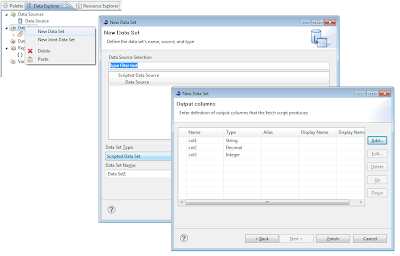
The final portion of this example requires that we add code to the script events to call the Java Excel API. Select the new data set and click on the script tab. From the drop down menu select the open event. Enter the following code.
importPackage( Packages.java.io);
importPackage( Packages.jxl );
try{
var inputWorkbook = new File(params["XLSLocation"].value);
myworkbook = Workbook.getWorkbook(inputWorkbook);
sheet = myworkbook.getSheet(0);
numrows = sheet.getRows();
curr = 0;
}catch(e){
curr=0;
numrows=0;
}
This code opens an xls file pointed to by the report parameter XLSLocation. You can hard code the file but this example is a bit more dynamic. The code then opens the first sheet in the workbook and sets a global JavaScript variable for the number of rows and initializes a current row counter to zero.
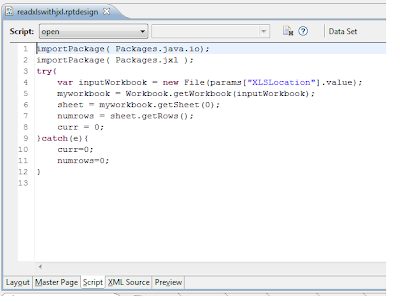
Select the drop down menu and select the fetch event and enter the following code.
if( curr < numrows ){
var cell1 = sheet.getCell(0, curr);
row["col1"] = cell1.getContents();
var cell2 = sheet.getCell(1, curr);
row["col2"] = cell2.getContents();
var cell3 = sheet.getCell(2, curr);
row["col3"] = cell3.getContents();
curr++
return true;
}else{
return false;
}
The fetch event is fired once per row of data and stops processing when the event returns false. In this code we are just retrieving the first three columns of the xls sheet and assigning them to the dataset row columns.
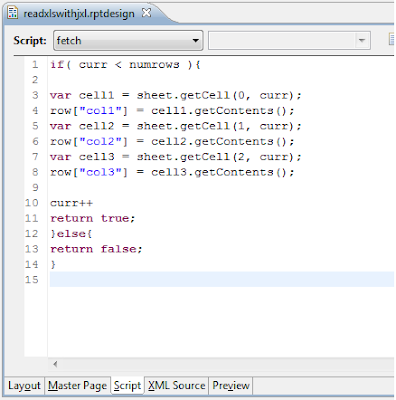
The final event to implement is the close event. Select close from the drop down box and enter the following code.
if( typeof myworkbook != 'undefined' ){
myworkbook.close();
}
This code closes the workbook.
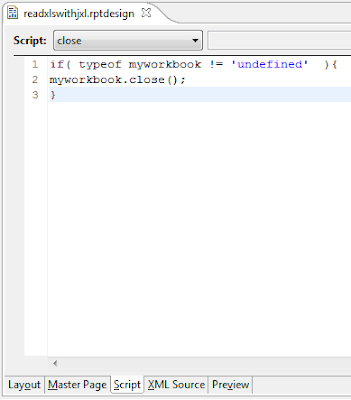
You should now be able to preview the dataset.
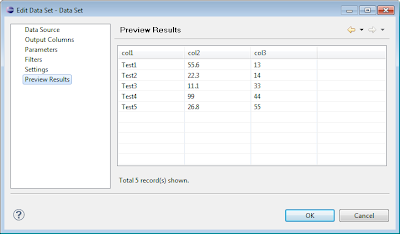
Remember to add the parameter that defines the location of the xls file. Once the dataset is complete you can construct your BIRT report as usual. This attached example produces the following report.
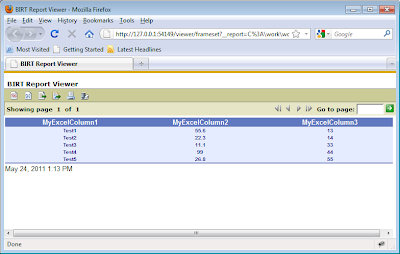
This example is available at BIRT-Exchange.