The Eclipse Test & Performance Tools Platform (TPTP) Project team has produced a very nice example of using BIRT to create reports based on profile information. You can see it here.
The report uses the new Chart Builder that was introduced in M3 as well as the new XML Data Source.
Sunday, December 18, 2005
TPTP and BIRT
Posted by
Jason Weathersby
at
11:38 PM
2
comments
Wednesday, December 07, 2005
Eclipse BIRT 2.0 Milestone 3 Released
The Eclipse Business Intelligence and Reporting Tools (BIRT) team has released Milestone 3 of BIRT 2.0. This milestone showcases some of the more lofty features of the 2.0 release, including library and template support, a new charting wizard, chart SVG output, a new XML data source and improved PDF and Report generation performance.
In addition to the features showcased in M3, progress on report paging, event debugging and scripting properties have made real progress. I have been building some examples to illustrate scripting and debugging and it is very cool.
The BIRT team is very interested in getting your feedback and suggestions on improvements.
To read about the feature set in BIRT 2.0 M3 take a look at and let us know what you think.
Notable BIRT 2.0 Milestone 3
Posted by
Jason Weathersby
at
6:32 PM
0
comments
Friday, December 02, 2005
Using a supplied connection with BIRT
In an earlier post, I said that I would try to use the new setAppContext feature within BIRT to implement connection pooling. Well in truth, I didn’t implement connection pooling but instead built an ODA that uses a supplied connection in place of the one BIRT would normally establish on its own. To understand where this would be useful, imagine that you have a J2EE application built that already has a connection pool set up. Ideally when your application calls a BIRT report, it would be nice if BIRT would use a connection that is already created. This is where the setAppContext method is very helpful.
A snippet of ReportRunner.java, which is part of the engine API and shows how to execute a report using the API, is shown below.
HTMLRenderContext renderContext = new HTMLRenderContext();
renderContext.setImageDirectory("image"); //$NON-NLS-1$
HashMap contextMap = new HashMap();
contextMap.put( HTMLRenderContext.CONTEXT_NAME, renderContext );
//Get a connection from the pool
testConn = setupJdbcConnection();
contextMap.put("org.eclipse.birt.report.data.oda.subjdbc.SubOdaJdbcDriver", testConn);
task.setContext( contextMap );
I have added two lines to this code, which are bolded. The first retrieves a java.sql.Connection object. In my example I created this connection directly. In other applications this would be returned from a connection pool call. The next line adds this Connection object to the context map. As the key for this map entry I am using the name of the intended recipient plug-in.
Similar lines of code would be added to an application that is using the engine APIs to call BIRT. Also note that task.setContext will change to task.setAppContext( Map) in BIRT 2.0 M3 and later.
Now my connection is within BIRT. The next step is to configure BIRT to use it. By default the setAppContext feature was created to allow application contextual information to be passed to the data layer. This normally would require an ODA to be built to use it. However there are other ways to get at and use this context. One way is to extend an existing ODA. The ideal choice is the JDBC ODA.
The JDBC ODA uses the OdaJdbcDriver class as the entry point to the ODA. So you may want to create a new Plug-in that extends this class.
public class SubOdaJdbcDriver extends OdaJdbcDriver
private java.sql.Connection passedInConnection;
public void setAppContext( Object context ) throws OdaException
{
HashMap ctx = (HashMap)context;
passedInConnection = (java.sql.Connection)ctx.get ("org.eclipse.birt.report.data.oda.subjdbc.SubOdaJdbcDriver");
}
public IConnection getConnection(String connectionClassName) throws OdaException
{
if( passedInConnection != null){
return new appContextDBConnection();
}else{
return new org.eclipse.birt.report.data.oda.jdbc.Connection();
}
}
.
.
.
The only methods we are concerned about are the setAppContext and getConnection methods.
As you can see the setAppContext method just retrieves the Connection set earlier in the caller code and stores it.
The getConnection method checks to see if the passedInConnection is not null. If it is null we want the BIRT framework to make the connection using the existing JDBC Connection class. This allows the report designer to continue to use standard BIRT connections at design time and while in run time it retrieves the connection from the calling applicaton.
The appContextDBConnection class is just an extended version of the JDBC ODA Connection class. This can be added as an inner class to the SubOdaJdbcDriver class.
private class appContextDBConnection extends org.eclipse.birt.report.data.oda.jdbc.Connection
In this class the only thing we are really interested in is overriding the open and close methods.
public void open(Properties connProperties) throws OdaException
{
jdbcConn = passedInConnection;
}
public void close( ) throws OdaException
{
if ( jdbcConn == null )
{
return;
}
jdbcConn.close();
jdbcConn = null;
}
The jdbcConn variable holds the java.sql.Connection object that is used to make the queries. So in the open method we just set that variable equal to the one that is passed in. The close method just nulls the connection, although ideally it would return the connection to the pool.
There is only one issue. The variable jdbcConn in the existing JDBC ODA is private. You have two choices, make a local version of jdbcConn and override all the methods that use this variable or change the BIRT source for the JDBC ODA to make jdbcConn protected. Post BIRT M3 this variable will be made protected.
If you choose not to change the BIRT source the following methods will have to be added to the appContextDBConnection class in addition to the open and close methods.
commit
getNaxQueries
getMetaData
isOpen
newQuery
rollback
The code for these methods should be exact copies of the methods in org.eclipse.birt.report.data.oda.jdbc.Connection class.
Remember to add the local copy of jdbcConn to the appContextDBConnection class.
private java.sql.Connection jdbcConn = null;
For this new plug-in copy the plugin.xml from the JDBC plugin and modify the driverClass line to point to the new SubOdaJdbcDriver class.
<datasource id="org.eclipse.birt.report.data.oda.subjdbc" odaversion="3.0" driverclass="org.eclipse.birt.report.data.oda.subjdbc.SubOdaJdbcDriver" defaultdisplayname="Example Context JDBC Data Source" setthreadcontextclassloader="false">
Also change the name, change the library tag and add the JDBC plug-in to the requires tag.
<plugin class="org.eclipse.birt.report.data.oda.subjdbc.plugin.SubjdbcPlugin" id="org.eclipse.birt.report.data.oda.subjdbc" name="Subjdbc Plug-in" version="1.0.0">
<runtime>
<library name="subjdbc.jar">
<export name="*">
</library>
</runtime>
<requires>
.
.
<import plugin="org.eclipse.birt.report.data.oda.jdbc"/>
</requires>
You will need to create a plug-in for the ui as well. This plug-in requires no code, just the plugin.xml
Copy the plugin.xml for the JDBC ui plug-in and change the odaDataSourceUI tag to point to the subjdbc plug-in.
<extension point="org.eclipse.birt.report.designer.ui.odadatasource">
<odadatasourceui id="org.eclipse.birt.report.data.oda.subjdbc">
Change the name of this plug-in as well. This plug-in exports no jar file and only need the JDBC ui plug-in to work.
<plugin class="" id="org.eclipse.birt.report.data.oda.subjdbc.ui" name="Ui Plug-in" version="1.0.0">
<requires>
<import plugin="org.eclipse.birt.report.data.oda.jdbc.ui"/>
</requires>
Export the two plug-ins and add them to your designer. Finally, add the subjdbc plug-in to the viewer plug-in and you are ready to develop reports that leverage supplied connections.
Posted by
Jason Weathersby
at
12:56 PM
29
comments
Monday, November 21, 2005
BIRT at EclipseCon
EclipseCon is the premier technical and user conference focusing on the power of the Eclipse platform. The BIRT project will be presenting a detailed half day tutorial on the extension and integration of BIRT. The presentation has been accepted and is on the EclipseCon schedule. As we begin the process of building the curriculum, we would be interested in any specific feedback on areas of interest that could be added to this presentation.
In addition to the advanced tutorial presentation, we are trying to get two additional one hour presentations about BIRT added to the EclipseCon schedule. Your votes and feedback can help us to spread the word about BIRT.
Introduction Talk - BIRT: The Eclipse Reporting Framework
Intermediate Talk - Leveraging BIRT Reporting in Your Applications
Posted by
Anonymous
at
10:18 AM
1 comments
Wednesday, November 16, 2005
Using BIRT To Report On Bugzilla in MySQL
MySQL has posted a good article on using BIRT to report on a Bugzilla MySQL database. The article covers some of the BIRT reporting capabilties, such as connecting to Bugzilla, nested grouping of bugs by product and component, and totaling.
Take a look at it at MySQL
Posted by
Jason Weathersby
at
2:01 PM
1 comments
Friday, November 11, 2005
Using Embedded Java Objects within BIRT
Embedded Java Object Reporting
Most often reporting tools pull data into the design tool or engine and then format, group and aggregate the data. The report developer fires up the design tool, creates the data sources and retrieves the result sets. The developer then proceeds to do the work of reporting. After completing the design, the report is usually deployed to some type of server. This model is usually sufficient for most applications.
In embedded applications this model does not always perform to expectations. for instance, assume that I have built an RCP application that handles benefits. The user of this application works through several data collection screens. At the completion of this task the user needs to print a summary of all the picks that have been made. As the choices were selected they are persisted to a database, so the natural inclination is to start up the report designer and report the choices from the database. This is not optimal for many reasons. If we already have all the choices the user made within a Java Object, I why would rather use it as the reports data source. Doing this would save at least one more trip back to the database. Some tools do this by allowing an Object type to be passed in as a parameter. In BIRT, this can be done in several different ways.
One of the more interesting and powerful ways is to use the addScriptableJavaObject method of the RunAndRenderTask class. This method allows the BIRT scripting engine to access the Java Object and use it as if it were native to the engine. This gives the application designer a lot of capabilities, such as passing in usernames, beans, and HTTPSession objects.
The method takes two parameters. The first is the name the script engine will reference the object by. The second is the actual Object instance.
In the case of our example, I add the BenefitObjectInstance as below.
//excerpt from the RunReport.java Example
IRunAndRenderTask task = engine.createRunAndRenderTask( report );
.
.
.
task.setParameterValues( params );
task.addScriptableJavaObject("BenefitObject", BenefitObjectInstance);
task.run( );
To use this object within the designer, I will reference the BenefitObject in script. I add a Data control and then place a reference to my Object in the Expression builder.
//Value for the Data Control
benefit =BenefitObject;
benefit.getSocialSecurityNumber();
In BIRT 2.0 an additional feature is being added that allows the application to pass in a full context object to the data layer. This will not only allow reporting off of existing Objects, but will allow things like credentials, parameters and connection handles to be passed in from the application. With BIRT’s M2 release, this feature is available now. I will write up a short example of how to use it in a later post.
Posted by
Jason Weathersby
at
2:47 PM
2
comments
Monday, November 07, 2005
Scapa and BIRT
Title: Scapa and BIRT
Scapa builds application performance testing, diagnosis and monitoring software. They currently use BIRT.
Neil Sanderson Scapa’s Development Manager provided the following explanation.
Scapa uses the BIRT reporting framework as a means of presenting its aggregated statistical data in a final, management-reporting-level format (HTML or PDF). Scapa's existing test-execution and monitoring tool controls the content of any report as well as many presentation options (graph-colors, vertical-scales and ranges) and then uses BIRT's own APIs to construct a BIRT-compliant, tabular data structure from which the final report document (containing tabular and/or graphical content) can be automatically generated.
You can get more information at http://www.scapatech.com
You can use the BIRT APIs to embed the report engine in to your application, allowing parameter collection, report generation and distribution.
To learn more about the BIRT Engine APIs take a look at
Using the Report Engine
Posted by
Jason Weathersby
at
2:07 PM
0
comments
Thursday, November 03, 2005
New BIRT Demo
The BIRT team has released a new BIRT Demonstration. This demo shows building a complex report against MySQL, illustrating several key BIRT functions such as grouping, highlighting, JavaScript in the Expression Builder, etc.
Here is a screenshot from the completed report.
Posted by
Jason Weathersby
at
2:18 PM
2
comments
Monday, October 31, 2005
BIRT 2.0 Milestone 2 Released
BIRT 2.0 Milestone 2 Released
The BIRT team has released Milestone 2 of BIRT 2.0. This milestone release as some very nice features such as dynamic and cascading parameters, importing style sheets, a new expression builder, stored procedure support, and BLOB/CLOB support.
BIRT 2.0 is shaping up to be a very cool release. Take a look at the features in milestone 2.0 at http://www.eclipse.org/birt/index.php?page=project/notable2.0M2.html and let us know what you think.
Posted by
Jason Weathersby
at
7:45 AM
0
comments
Thursday, October 27, 2005
Linux BIRT Server
John Ward over at the Digital Voice http://digiassn.blogspot.com, has posted two very good articles on setting up a Linux BIRT report server. The articles walk through a complete server setup, which uses Tomcat, wget, and Cron. Some of the key areas covered are publishing, deploying and even scheduling BIRT Reports.
John, thanks for the articles.
Part 1
http://digiassn.blogspot.com/2005/10/birt-report-server-pt-1.html
Part 2
http://digiassn.blogspot.com/2005/10/birt-report-server-pt-2.html
Posted by
Jason Weathersby
at
11:58 AM
8
comments
Using BIRT on the iSeries
Using BIRT on the iSeries (Formerly IBM AS/400)
One of our active posters within the BIRT news group, who goes by "RAM" posted this article on accessing iSeries data. It is very nice when users in the community take the time to help others solve problems.
This document shows how to get BIRT up and running on the iSeries.
Step 1: If you do not have access to an iSeries machine, download JT400 from http://jt400.sourceforge.net/ and unzip it to a folder on your PC. For example, assume that you unzipped the file into c:\JT400. .
Step 2: Inside this folder you will find another folder named LIB. IN this folder, you will find a file named JT400.JAR. This file contains the JDBC drivers. If you have access to an iSeries machine, you can find the file in folder /qibm/proddata/HTTP/Public/jt400/lib.
Step 3: Download the BIRT Report Designer from:
http://www.eclipse.org/downloads/download.php?file=/birt/downloads/drops/R-R1-1_0_1-200508091640/birt-rcp-report-designer-1_0_1.zip.
Step 4: Unzip the BIRT report designer into a folder. Assume that this folder name is C:\BIRTDesigner
Step 5: To design a report, double click the file named BIRT.EXE in the above folder.
Step 6: Click on FileNew Report.
Step 7: Type in a name for the report, click Next, select style and hit Finish.
Step 8: Right click on “Data Sources” and select “New Data Source”. In the following window, select JDBC Data source and click Next.
Step 9: The new data source window will appear as shown.
Step 10: Click on the Manage Drivers button. This will bring up the Manage drivers window as shown below.
Step 11: Click on Add, browse to the folder containing the JT400.JAR file and click on Open. This will add the JT400.JAR file as shown below. Hit OK.
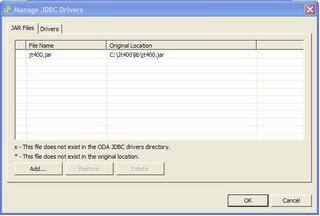
Step 12: On the New Data source window, use the following:
Driver Class = com.ibm.as400.access.AS400JDBCDriver
Database URL = jdbc:as400://as400SystemName
User Name = your user name
Password = Your password.
You can test the connection using the Test Connection button.
This should get you up and running in trying to design the report on the PC.
Setting up the server:
Step 1: Download and install Tomcat on the iSeries. The instructions are available at the following link:
http://www.itjungle.com/mpo/mpo021402-story02.html
Instead of using Tomcat 4.0.1 as stated by David Morris, just use Tomcat 5.0.28.
Step 2: Download and unzip BIRT Runtime on to your PC from http://www.eclipse.org/downloads/download.php?file=/birt/downloads/drops/R-R1-1_0_1-200508091640/birt-runtime-1_0_1.zip.
Step 3: Once the file is unzipped, look for the file named birt.war in the unzipped folder. FTP this file to the iSeries into folder /tomcatInstallationFolder/WEBAPPS, where tomcatInstallationFolder is the name of the folder where you installed Tomcat.
Step 4: Re-start Tomcat. That should do it.
Once your design is complete, upload your .rptdesign file into the /tomcatInstallationFolder/webapps/birt folder.
Then use the link:
http://iSeries:portnumber/birt/frameset?__report=filename.rptdesign
Where filename is the name of the report design file.
Posted by
Jason Weathersby
at
10:33 AM
0
comments
Monday, October 24, 2005
Zend and BIRT
As you know, PHP is the technology of choice for many web site developers because of its simplicity and power. PHP applications, be they CRM applications, e-commerce applications, performance reports or help desk applications, usually require reports. However, even with PHP, creating reports for a PHP based web application can be tedious (Examples: a sales invoice, or a sales commission report). Yes, the first one is not too tough to do, but then you decide you need a second report, then a third -- and before you know it you are building a whole reporting product in PHP.
So, what is the solution?
Well, Zend and the Business Intelligence and Reporting Tools (BIRT) team are working together to combine the simplicity and power of PHP for your web site, with the simplicity and power of BIRT for your reports. The goal is to allow the PHP developer to easily add sophisticated reports to the feature rich PHP environment. While the details are still TBD, the teams are looking at creating PHP classes to easily run and view BIRT reports from within the PHP language.
Watch this space.
Posted by
Jason Weathersby
at
3:24 PM
6
comments
BIRT on Tech Forge
Just want to give a tip of the hat to my fellow blogger, Jason Weathersby on the publication of an article on Tech Forge that demonstrates how to integrate BIRT with Hibernate through a JavaScript data source.
For those of you interested in using BIRT with Hibernate, the article provides a great starting point for a quick and not too dirty implementation of Hibernate with BIRT. Eventually, we will be providing an implementation of a Hibernate data source using BIRT, but that will probably have to wait until we get through the 2.0 release cycle.
Scott
Posted by
Anonymous
at
2:35 PM
0
comments
Wednesday, October 19, 2005
BIRT Examples
We are currently in the process of revising the examples section of the BIRT site.
Take a look and let us know what you think. This list of examples will grow, so any suggestions or submissions are appreciated.
Posted by
Jason Weathersby
at
2:44 PM
134
comments
Friday, September 30, 2005
BIRT 2.0 Project Plan
The BIRT team has released the Project Plan for BIRT 2.0, which will include features like page on-demand navigation, progressive viewing, table of contents support, library usage, external css files, improved scalability,... This list goes on. For the full list of planned features see the Eclipse BIRT site.
Posted by
Jason Weathersby
at
2:39 PM
0
comments
Sguil Event reporting with BIRT
John Ward has posted a very good article on Sguil reporting, using BIRT.
You can read about it at The Digital Voice. The article shows quite a few features of BIRT including things like highlighting.
Posted by
Jason Weathersby
at
7:26 AM
0
comments
Friday, September 09, 2005
Report Functional Segregation
On the BIRT message board, I was asked, “isn't it better and make much sense to split the rpt design into two pieces:- one that contains all the screen layout details (the actual view part) and another part that contains the actual business logic and the query?”
One of the core designs in BIRT is that the ROM allows report developers to do just that. The breakdown of what goes where may not be what you were expecting, but it does have a logical break out of functionality. One of the key tasks for BIRT developers will be deciding where they should place their unique custom logic to achieve the maximum functionality and reuse.
So how should logic be split up in a BIRT report? Well like many I am still exploring the functionality in BIRT, and I am still waiting to see how the Library functionality is applied in BIRT 2.0. That said, I approach report designs thinking of five key functional areas. The links on the component names will take you to the RomDoc on the BIRT web site which is a valuable resource for understanding what a component can do.
Report Specific Properties (ReportDesign )
Data Acquisition and Preparation (DataSource, DataSet, Row, Parameter)
Report Structure (Grid, Table, TableGroup, Row, Column, )
Report Format (Style, TextData, Text, Image, Rectangle, Label, Line)
Page Presentation (GraphicMasterPage, SimpleMasterPage)
When libraries are enabled in 2.0 this breakdown of functionality will really start to be important. With libraries BIRT developers will be able to create sophisticated report components that can be re-used in multiple report designs.
For example, the DataSource component is responsible for a connection to the outside world that is used to acquire data. A good DataSource component would be able to query the run-time environment, perhaps for a configuration file, to determine what the DataSource should connect to, Dev database in the Dev environment, stageDb in the Stage environment, etc.
In my next few posts, I will attempt to spend a bit more time discussing the various types of components and how they are logically combined and used.
Posted by
Anonymous
at
6:50 AM
0
comments
Friday, September 02, 2005
BIRT 1.0.1 Feature Set
Eclipse Business Intelligence and Report Tools (BIRT) Project
Introduction
BIRT is an Eclipse-based open source reporting platform for web applications, especially those based on Java and J2EE. BIRT has two main components: a report designer based on Eclipse, and a runtime component that you can deploy to your application server. BIRT also has a charting engine that lets you include charts in your BIRT reports or add standalone charting capabilities to Java applications.
Report Designer
Application development with BIRT starts with the report designer. This Eclipse-based interface offers an HTML page-oriented design metaphor (similar to Adobe Dreamweaver and other HTML editors) to build reports that are intuitive to create and integrate easily into web applications. Key components include:
- Data Explorer – Manages your data sources (connections) and data sets (queries).
- Layout View – WYSIWYG editor that provides drag and drop creation of the presentation portion of your report.
- Report Item Palette – A palette with a rich set of visual elements such as containers (tables, grids, lists), labels and others.
- Expression Builder – Provides an interface to create calculated fields, format existing ones or perform other data-oriented calculations.
- Style Builder – Creates and manages CSS-like styles for consistent formatting.
- Property Editor – Presents the most commonly used properties in a convenient format that makes editing quick and easy.
- Report Preview – Allows you to test your report at any time with real data.
- Code Editor – Edits custom business logic to be executed during data access, report generation, or viewing.
- Chart Wizard – Defines and formats charts and their accompanying legends and axes.
- Outline – Provides a compact overview of your entire report structure.
- Cheat Sheets – Offer step-by-step instruction on commonly performed tasks to shorten the learning curve.
BIRT provides two flavors of designer: a plug-in for the Eclipse IDE, or a Rich Client Platform (RCP) version that offers a simplified interface without the additional perspectives in the standard Eclipse platform.
Report Design File
The report design file, as expressed by the BIRT Report Object Model (ROM), is the key design “artifact” associated with a report. It contains the elements that make up a report, including visual design components such as a table or an image and other non-visual items such as report parameters, formatting styles and data sources.
The designer allows the user to define these elements, the design file stores the elements in an XML format adhering to the ROM, and the report engine interprets the elements to produce a report document as output.
Report Engine and the BIRT Viewer
The BIRT report engine API allows you to integrate the run-time part of BIRT into your application as a set of POJOs (Plain Old Java Objects). The engine enables you to discover the parameters defined for a report and get their default values, incorporate included images or charts, retrieve needed data and transform it as necessary and, finally, render the report in HTML or PDF. Report execution also encompasses execution of any custom business logic associated with the report that is written in JavaScript or Java.
Included with the BIRT distribution is a sample BIRT viewer. It is used within the report designer for the preview operation, but can also be used as a simple user interface in your Java application to prompt for report parameters prior to report execution via the report engine API.
Chart Engine
The chart engine provides a rich business chart generation capability to be used standalone or invoked from the report engine. The chart engine supports creation of pie, bar, line, scatter and stock charts, all of which can be rendered in the following graphic formats: 8-bit images, 24-bit images, SWT graphics (GC), SWING graphics (Graphics2D) and SVG.
Design Engine API
The BIRT Design Engine API (DEAPI) enables the programmatic creation or modification of report designs and also provides for interrogation of report elements and semantic checking.
Extensibility
BIRT is more than a reporting tool; it is an extensible reporting framework. As such, it provides a number of extension points to support application-specific and general-purpose modifications. These include:
- The Open Data Access (ODA) framework, which enables the addition of new data sources to BIRT. It provides for both a runtime driver and a design time user interface.
- Custom scripting to incorporate complex and/or application specific business logic into report designs.
- APIs that permit the introduction of new visual components into reports and new attributes for existing components.
- Pluggable architecture for incorporating custom charts and new graphic formats into the charting engine.
Internationalization
BIRT is Unicode enabled, and supports creation of reports in any locale, with the exception of Right-to-Left languages.
Posted by
Jason Weathersby
at
8:41 AM
39
comments
Friday, July 15, 2005
Welcome to BIRT World
The Eclipse Business Intelligence and Reporting Tools project (BIRT) is a top level project of the Eclipse Foundation. This BLOG will be focused on the use and issues associated with the BIRT project.
To start here are a few links to some other great BIRT resources.
Home Page The BIRT Home web site
News Server The BIRT News Group
Web Interface For those of you that do not use Newsreaders
More to come soon,
Scott Rosenbaum
BIRT PMC
Posted by
Anonymous
at
10:46 AM
0
comments