Recently I had the opportunity to figure out how to manipulate sizing, positioning and text wrapping of chart legends so I thought I'd share what I learned.
If you have a chart with legend items that don't fit in the available space, BIRT will do one of two things, depending on the wrapping width option. (Wrapping width is found on the legend layout dialog). If the wrapping width is set to zero, BIRT will simply truncate the legend item text and optionally append an ellipsis. (The ellipsis option is located on the legend entries dialog).
If the wrapping width is set to a positive value, BIRT will word-wrap the text. Unfortunately when it does this, it doesn't check the vertical boundaries and long items can end up overlapping.
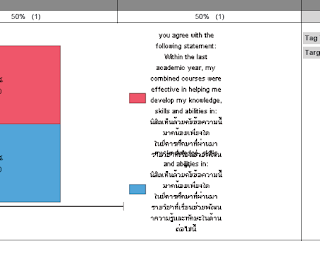
It turned out I could do a lot, but I had to pick the right event handler. My first thought was to use beforeDrawLegendItem. It looked perfect because of the Bounds parameter. However, it turned out that was not the bounds of the text area, but of the colored rectangle, which didn't help me much. Additionally I couldn't manipulate the size and position of the legend items within that handler and have it stick. The horses had already left the barn so to speak.
The beforeRendering handler was much better. In it I could manipulate the size, position and text of each legend item. Following are the the technical details.
The first argument to the event handler function is a GeneratedChartState object (which I'll call gcs). Using gcs.getRunTimeContext(), you can get the RunTimeContext object (rtc). Then using rtc.getLegendLayoutHints() you get the LegendLayoutHints object (llh) which tells you about the legend as a whole. Particularly llh.getLegendSize() tells you how big BIRT wants to make the legend. Finally llh.getLegendItemHints gets you an array of LegendItemHints (liha), which gives you access to each legend item. The number of legend items is liha.length. The text for each item is lih.getItemText().
So how much height is available for the whole legend? You can get that with gcs.getPlotComputations().getPlotBounds().getHeight(). The width of the legend is llh.getLegendSize().getWidth(). I didn't need to change the width so I didn't try to find out how to do that. I'm not sure how BIRT allocates horizontal space to the legend vs the chart itself. That may present itself as a future exercise.
For my project I had to wrap this text according to the space that was available, so I needed to be able to measure the pixel width of a string of characters. To do this you need to instantiate BIRTChartComputation. The constructor takes no arguments so it's simple. I'll call my instance bcc. Next you need the font height, which you can get from bcc.computeFontHeight. This method needs an IDisplayServer and a Label. You can get the IDisplayServer from gcs.getDisplayServer() and you can create a temporary Label with GObjectFactory.instance().createLabel(). The method with compute the font height based on the text in the label. Finally, you get the overall size of a string of text with bcc.computeLabelSize, which you pass an IDisplayServer, a Label and a font height. This is a fairly expensive operation so it's best to use a binary search to look for a wrap point.
Once a new version of the text has been computed, you can simply insert it back into the LegendItemHint using lih.setItemText(). Also the item height can be set with lih.itemHeight(). Finally, if you want an ellipsis to be appended you need to set the valid item length with lih.validItemLen() to the character position where you want the ellipsis to go. If you set it to zero or a value greater than the length of the label, no ellipsis will be appended.
Since I also needed to change the overall size of the legend, I wanted a setLegendSize method in LegendItemHints, but no such method exists. In fact, the class is mostly immutable. Fortunately it's possible to instantiate a new LegendItemHints with a new size and pass it to the RunTimeContext with rtc. setLegendLayoutHints().
An that's it. Fun, huh? Ok you can stop yawning. Here's what my new legend looks like:
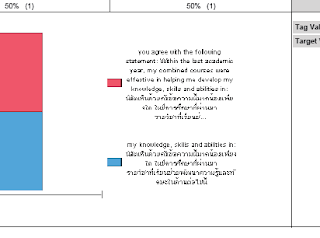
2 comments:
In Case for Horizontal wrapping of dynamic legends u can use the following property:
From your Eclipse designer: set Format Chart->Legend->Entries->Ellipsis property to possible max length of your dynamic legends. and the problem will be solved.
As this property work as follows:
The default value is "1". Int attribute "Ellipsis" specifies the behavior of shortening the legend item's text with ellipsis if there is not enough space to display the whole text. Value 0 indicates that the feature is disabled, and the legend item will either be displayed with whole text or be dropped. A positive value n represents the minimal count of characters to be displayed before the ellipsis, which means the legend item will either be dropped or be displayed with at least n characters.
Hope this will solve your problem. It worked for me.
Great rreading
Post a Comment